At qAPI, we’re focused on one mission: simplifying API testing so that teams can move faster, debug smarter, and release more with confidence. This will in turn increase productivity when it comes to functional API testing.
We’ve seen a clear pattern emerge across hundreds of engineering teams: writing API test cases takes too long and debugging them across multi-step workflows is even harder. It’s not just a developer frustration—it’s a managerial setback that’s affecting delivery timelines and system stability.
In 2024, 74% of respondents are API-first, up from 66% in 2023, with an average application running between 26 and 50 APIs actively. This shift toward API-first development has created new testing challenges.
Failing to complete digital transformation initiatives is costing organizations a minimum of $9.5 million annually, largely due to integration failures and inadequate API testing. And these numbers are small if we focus on the largely affected aspects, if we zoom out and look at the big picture, the number will be bigger.
As part of this collective strategy, we have launched our functional API testing tool, which helps you create test cases with ease in the cloud.
We understood the setbacks teams face with the current tools on the market and created a way to leverage AI to reduce the time wasted in running behind a manual testing process.
Here, we’ll take a closer look at what qAPI’s API testing capabilities are, how they work, and how they’ll help teams save time and make the most out of their API testing needs.
Let’s clear the basics first.
What is Functional API Testing and Why is it Important?
Functional API testing is the process of verifying that an API performs as per its defined functions correctly, meeting its specified requirements.
It can be many things like sending requests to API endpoints and checking if the responses align with expected outcomes, including correct data, proper error handling, and follow specifications.
Unlike performance or security testing, functional testing focuses on the API’s core functionality—making sure that it does what it’s supposed to do under any condition.
Importance of Functional API Testing
A single API failure, if not tested and identified early can lead to infamous issues, such as:
• Data Breaches: Improper handling of authentication or authorization, which exposes sensitive data.
• Service Disruptions: Faulty APIs will cause spiralling failures across dependent systems.
• Poor User Experience: Incorrect responses or slow performance will result in the loss of more customers and visitors.
Functional API testing ensures reliability, security, and performance, which are important for maintaining user trust and application likeability.
To create a good and scalable API testing framework, you and your team needs to identify the key areas of performance that will be used as a reference point to test APIs.
The Market Gap
Let’s just pick the trending markets — a typical e-commerce checkout process now involves 25-30 API calls across authentication, fraud detection, inventory management, payment processing, tax calculation, shipping logistics, and order confirmation.
If each step is connected to the previous one, and any failure can affect the entire workflow. That’s why studies have shown that 68% of API failures occur in multi-step workflows rather than single endpoint calls.
The problem? Most API testing tools are still designed to validate individual endpoints, rather than creating complex workflows.
This is what qAPI solves.
qAPI’s Functional API Testing capability is designed to solve these exact issues. Here’s how:
• Import any API collection (Postman, Swagger, etc.) and instantly generate workflow-based test cases
• Customize flow logic, with chaining, conditions, retries, and validations
• Run functional and performance tests together—one click, two test types
• Debug faster, with AI-driven test case generation and reporting insights get recommendations and solve issues faster.
• Automate API tests 24×7
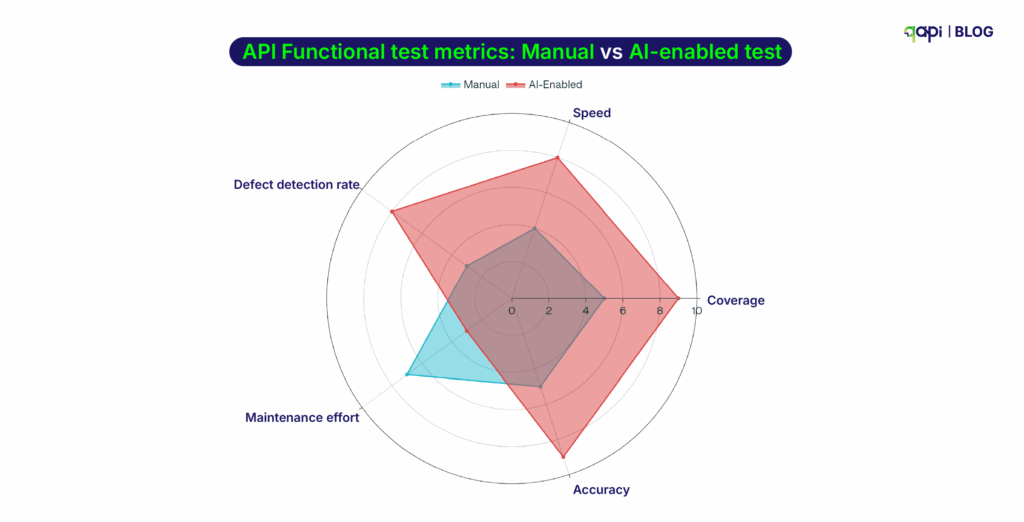
Based on current growth trends and enterprise adoption rates, we project that by 2027, organizations will manage an average of 75-100 APIs per application, driven by increased adoption of microservices and third-party integrations. This shows a 50% increase from current levels.
What challenges should I expect in functional API testing?
Because when it comes to managing environments, there’s still a problem.
APIs Change Fast. Tests Don’t Keep Up.
APIs will change—new versions will come so will new endpoints, and changed fields. But with every change, your test suite needs to be updated too, which includes: test data, environment setup, and validation rules.
Every API version you support requires additional effort to maintain: adjusting test data, assertions, and environments. A systematic review highlights ongoing struggles with “authentication-enabled API unit test generation,” showing major maintenance gaps
Example: When your /user/profile endpoint changes to return an extra nickname field, old tests expecting only name may silently break or miss validation. Over time, many tests become outdated.
And yet, most legacy testing tools—like Postman or Swagger-based setups—are still focused on one endpoint at a time. They weren’t built to test connected workflows or simulate production-like sequences.
Most tools don’t handle this well. The result? Teams start ignoring broken tests—or worse, they stop writing them altogether.
Also, There Are Multiple Slow Feedback Loops
API tests that take 20 minutes to run don’t help developers. By the time you get results, you’ve moved on to other tasks. Fast feedback is crucial for modern development workflows. Manual testing is a slow road. API tests should run automatically on every pull request or build.
Tests Are Not Integrated Into CI/CD
Only 30% of teams today automate Postman tests in their CI/CD pipelines. Many still run them post-deployment. That’s too late.
In fast-moving development cycles, feedback loops need to be short. If your tests take 20 minutes, your developers have already moved on.
This needs to change and to break this cycle, testing tools must follow these steps:
Best Practices for Functional API Testing in 2025
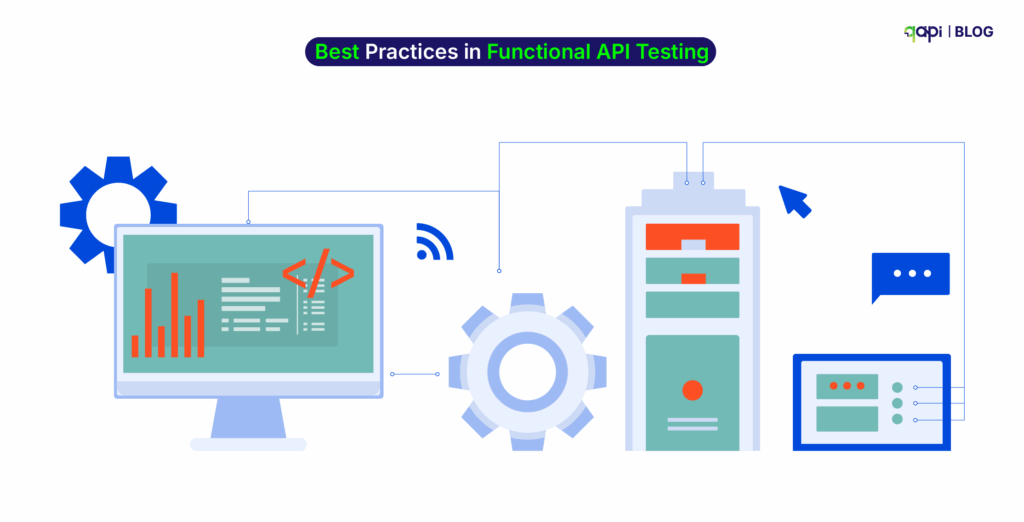
To ensure effective functional API testing in 2025, start doing these API testing best practices tailored to the latest technological advancements:
1️⃣ Integrate Testing Early in Development Begin testing during the development phase to identify and fix issues before they escalate. Early testing reduces costs and ensures quality from the start.
2️⃣ Use API Mocking and Simulation Tools like qAPI for virtual user simulation or Postman Mock Servers for testing without relying on real backend services, reducing dependencies and speeding up cycles.
3️⃣ Automate Regression Testing Automate regression tests to ensure new changes don’t break existing functionality. This is crucial for maintaining consistency in fast-paced development environments.
4️⃣ Validate HTTP Status Codes and Error Handling Verify that APIs return correct status codes (e.g., 200 OK, 401 Unauthorized) and handle errors gracefully to maintain application stability.
5️⃣ Integrate Tests into CI/CD Pipelines Automate tests within CI/CD pipelines using tools like Jenkins or GitHub Actions to ensure every code change is tested.
• Add test triggers in your CI pipeline (e.g., GitHub Actions, Jenkins, GitLab).
• Run smoke tests on every PR, deeper tests nightly or before release.
• Generate test reports and alerts automatically.
6️⃣ Leverage AI for Testing AI-driven tools can generate test cases, identify vulnerabilities, and predict failures based on historical data. By 2025, 40% of DevOps teams are expected to adopt AI-driven testing tools, enhancing efficiency and reducing errors.\
7️⃣ Choose Tools That Match Your Workflow
Not every tool suits every team. Choosing based on popularity rather than fit often leads to rework and frustration.
Choose tools that support your auth, CI/CD, and API types (REST, GraphQL, gRPC).
Evaluate whether it can scale with test volume and handle async operations.
Ensure your team can learn and maintain it quickly.
Examples:
Postman: Best for simple REST tests and manual workflows.
REST Assured: Good for Java-based validation-heavy use cases.
Karate: Great for BDD-style test writing and CI automation.
qAPI: Cloud-native, AI-powered, adapts to any workflow, it’s built to automate both functional + performance testing workflows in one place.
8️⃣ Start to Validate Error Handling
• Test invalid inputs, missing fields, bad tokens, and unsupported methods.
• Validate that error messages are clear and HTTP status codes are correct.
• Simulate failures in dependent services to test recovery logic.
Gartner estimates that 31% of production API incidents are due to poor error handling—not code bugs.
Best Practice | Description | Tools/Techniques |
Start Early | Test APIs during development, not after | qAPI with any other tool |
Mock APIs | Use simulators to avoid backend dependencies | Postman Mock Server, qAPI |
Automate Regression | Validate that updates don’t break old features | qAPI, CI pipelines |
Validate Status Codes | Ensure proper HTTP codes and responses | All major tools |
CI/CD Integration | Trigger tests on PRs, builds, or nightly runs | GitHub Actions, Jenkins, GitLab |
AI-Powered Testing | Generate, maintain, and debug tests with AI | qAPI |
Choose the Right Tool | Align tools with your stack and workflows | qAPI |
Test Error Handling | Simulate bad inputs, broken auth, failures | qAPI |
How can I automate functional API tests effectively?
Just bring your collection to qAPI.
1️⃣ Import your Postman or Swagger files.
2️⃣ Create a dedicated workspace.
3️⃣ Let our AI generate intelligent test cases.
4️⃣ Schedule or run tests immediately.
5️⃣ Track, debug, and optimize—on the cloud.
And that’s it. Here’s a video that takes you through it
Apart from API testing, Qyrus offers a single platform for automating a wide range of testing types, including:
• Cross-browser testing
• Mobile testing
• Web testing
• SAP Testing
Qyrus is not just an API testing tool—it’s a comprehensive, AI-driven testing platform designed to streamline quality assurance across the board. It offers a wide range of testing solutions application.
The Future of API Testing: What the Latest Data Tells Us
The API economy is no longer emerging—it’s exploding. And the numbers confirm it. If you’re still testing APIs like it’s 2018, you’re already behind.
Here’s what our most recent research reveals—and why it matters to your functional testing strategy:
API Usage Is Increasing
Treblle’s independent study of 1 billion API requests from 9,000 APIs found that APIs accounted for 83% of all internet traffic
Microservices Are Multiplying Rapidly
As per the CNCF 2024 Annual Survey, a typical enterprise runs 200–500 microservices, each exposing 2–3 APIs.
That’s anywhere between 600 to 1,500 APIs per organization—and each API must be tested for version compatibility, functionality, and chained workflows. Manual or endpoint-level testing is simply not logical in this scenario.
A recent forecast by IDC states that by 2027, 60% of enterprise development teams will rely on AI-assisted or fully autonomous testing tools.
Similarly, Gartner predicts that 85% of customer interactions will occur via APIs—not front-end channels—by the same year.
APIs now are the primary customer interface, and test coverage will need to evolve from manual scripting to AI-powered automation for teams to keep up.
Put all this together, and the message is clear:
• API volume is rising fast
• Functional complexity is increasing
• Existing tools can’t scale to handle dynamic workflows
• Test gaps are costing real money
• AI will be the only sustainable way to manage testing velocity and coverage
• Workflow-centric validation
• Integrated performance + functional test execution
And that’s exactly what qAPI is delivering.
What’s your biggest API testing challenge?
Share your experiences in the comments below, and let’s build a community of practitioners who can learn from each other’s successes and struggles.
For more insights on API testing best practices, subscribe to our newsletter and download our comprehensive API testing checklist to ensure you’re covering all the essential aspects of functional API validation.
FAQ
Use tools like Postman and qAPI to script end-to-end API calls. Next connect your requests by passing data from one response to the next and automate execution in your CI/CD pipeline for regular validation.
It is always a good practice to store test data separately from test scripts. Use environment variables for dynamic data and reset or clean up data before and after tests to ensure consistency and repeatability.
Automate token generation or use environment variables to store credentials securely. Then include the authentication steps in your test setup so that every test runs with valid access.
Use mocking tools like qAPI to simulate different responses, including errors and delays. This lets you test how your API handles failures without relying on real third-party services.
Top tools include Postman and qAPI. Choose based on your tech stack, scripting needs, and integration with your CI/CD workflow. It is also recommended to use both to save time and reduce code-based complexity.
Maintain test suites for all supported API versions and run them against each release. Communicate changes clearly and remove old versions slowly to avoid breaking existing clients.
Start by setting up tests and wait for callbacks, poll for results, or listen for webhook events. Use timeouts and retries to handle delays, and confirm the final state or response once the event is received.
Our teams regularly review and update tests to match their API changes. Utilize version control, clear documentation, and modular test design to simplify updates and minimize maintenance effort.
Send invalid, missing, or boundary data in your requests to trigger errors. Now, check if the API returns correct status codes and messages for each scenario.
Use data-driven testing to cover multiple input scenarios. Validate both the structure and content of responses, and use assertions that account for expected variations in data.